1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157
| function Person(first, last) { if ( arguments.length > 0 ) this.init(first, last); } Person.prototype.init = function(first, last) { this.first = first; this.last = last; } Employee.prototype = new Person(); Employee.prototype.constructor = Employee; Employee.superclass = Person.prototype; function Employee(first, last, id) { if ( arguments.length > 0 ) this.init(first, last, id); } Employee.prototype.init = function(first, last, id) { Employee.superclass.init.call(this, first, last); this.id = id; } ```
可以看到,我们把初始化属性的操作提取出来放到了一个init函数中,这样做是为了方便在子类中调用。注意其中的"**Employee.superclass = Person.prototype;**",这里是一个技巧,方便下面在子类中调用父类的方法。 另一个需要注意的地方是"**Employee.superclass.init.call(this, first, last);**":对于所有对象中的方法来说,都可以通过两个方法来调用--"**call**" 和 "**apply**". 这里使用了**call**方法,其中第一个参数是将在调用的方法中访问的对象,后面的参数与调用方法的参数一致。 **apply**方法的使用与call大致一致,不同在于除了第一个参数外,后面是一个参数数组。
下面附上完整的例子和类图:
继承关系图如下:
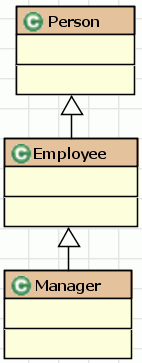
代码: ```javascript
function Person(first, last) { if ( arguments.length > 0 ) this.init(first, last); }
Person.prototype.init = function(first, last) { this.first = first; this.last = last; };
Person.prototype.toString = function() { return this.first + "," + this.last; };
Employee.prototype = new Person(); Employee.prototype.constructor = Employee; Employee.superclass = Person.prototype;
function Employee(first, last, id) { if ( arguments.length > 0 ) this.init(first, last, id); }
Employee.prototype.init = function(first, last, id) { Employee.superclass.init.call(this, first, last); this.id = id; }
Employee.prototype.toString = function() { var name = Employee.superclass.toString.call(this); return this.id + ":" + name; };
Manager.prototype = new Employee; Manager.prototype.constructor = Manager; Manager.superclass = Employee.prototype;
function Manager(first, last, id, department) { if ( arguments.length > 0 ) this.init(first, last, id, department); }
Manager.prototype.init = function(first, last, id, department){ Manager.superclass.init.call(this, first, last, id); this.department = department; }
Manager.prototype.toString = function() { var employee = Manager.superclass.toString.call(this); return employee + " manages " + this.department; }
|